CS488 Final Project - Ray Tracer
A questionably curated collection of pixels.
As part of CS488 - Intro to Computer Graphics, I have created a final project as an extension of the simple ray tracer created in assignment four. For this project, we were to create our own list of ten objectives and implement them. Mine are as follows.
Objective zero: Mirror Reflections
For assignment four I implemented mirror reflections as my extra feature, by recursively raycasting a ray created using glm::reflect()
Objective one: Torus and Cylinder primitives
In addition to the spheres and cubes of assignment four, I have added uncapped (but finite) cylinders and torii.
Objective two: Soft Shadows
For this objective, I introduced area lights by casting multiple shadow rays from each interseciton point. These rays were randomly perturbed from the original shadow ray direction.
Objective three: random supersampling
To smooth "jaggies," functionality was added to cast multiple rays through the sub-pixels of each pixel and average the results.
Objective four: glossy reflections
Implemented by randomly perturbing multiple reflection rays.
Objective five: Phong shading
Instead of using face normals, a mesh's vertex normals are interpolated using barycentric co-ordinates.
Objective six: texture mapping
Cubes and spheres have had UV co-ordinate calculations made available. These UVs are used with bilinear interpolation to select the proper pixel from the texture.
Objective seven: depth of field
Implemented by distributing ray origins along a 10x10 "square lens," setting direction such that they all intersect at the focal length.
Objective eight: refraction
Similar to reflecton, implemented by recursively tracing rays refracted according to Snell's laws via glm::refract.
Objective nine: bump mapping
Using the UV calculation from texture mapping, I use the retrieved colour value to perturb the surface normal of the primitive.
Objective ten: final scene
Rendered in ~three hours by GL11, supersampling is turned off as depth of field fills the same role here. Glossy reflections are also turned off for the sake of time.
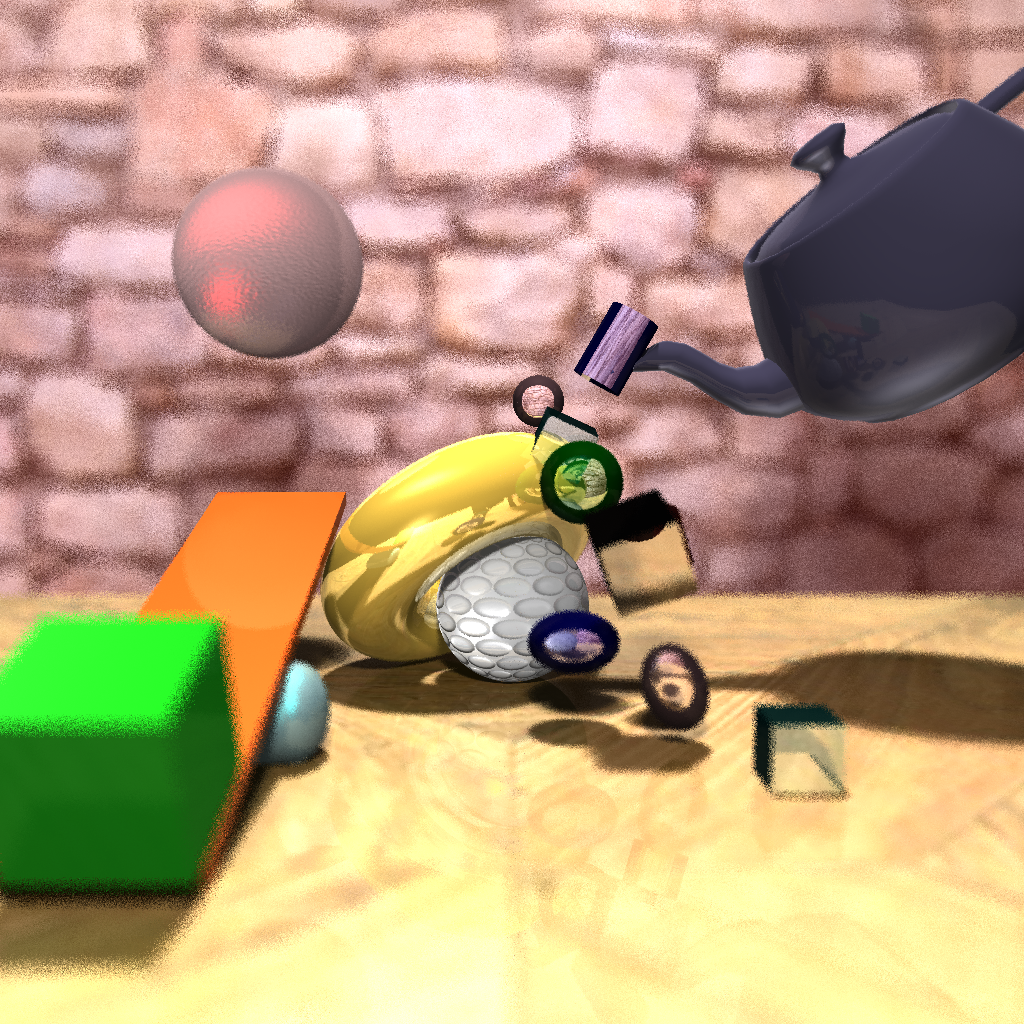
Extra objective: multithreading
This was done by breaking the image into a number of parallel chunks. Rendered using my final scene on gl13 at 512x512, with most extensions disabled.